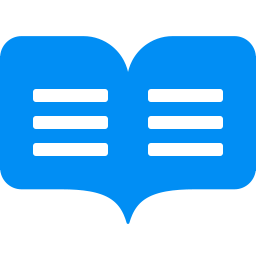
Vouchery.io
ShoppingExploring the Vouchery API Documentation
If you're looking to integrate Vouchery's services into your web or mobile applications, you're in luck. Vouchery provides a set of APIs that enables developers to programmatically interact with their platform. In this blog post, we'll explore the Vouchery API documentation and look at some example code in JavaScript.
Overview of the Vouchery API
Before diving into the specifics of the API, let's take a quick look at what Vouchery is. Vouchery provides a platform that enables businesses to create and manage targeted voucher campaigns. With the Vouchery API, developers can programmatically create and manage voucher campaigns, track voucher redemptions, and more.
The Vouchery API is organized into several resources, including:
- Accounts: Manage Vouchery accounts
- Campaigns: Create and manage voucher campaigns
- Customers: Manage customer data
- Vouchers: Issue, redeem, and manage vouchers
Each resource in the API provides a set of endpoint URLs that developers can use to interact with the Vouchery platform.
Getting Started
To get started using the Vouchery API, you'll first need to sign up for an API key. Once you have an API key, you can use it to authenticate your requests to the API.
const apiKey = "<your_api_key>";
fetch("https://api.vouchery.io/v1/accounts", {
headers: {
Authorization: `Bearer ${apiKey}`,
},
})
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error));
In this example code, we're making a GET request to the https://api.vouchery.io/v1/accounts
endpoint and passing the API key in the Authorization
header. The response is then logged to the console.
Example API Requests
Here are some example API requests that demonstrate how to interact with the Vouchery API using JavaScript.
Create a Campaign
const createCampaign = async () => {
const apiKey = "<your_api_key>";
const campaignData = {
name: "New Campaign",
start_date: "2022-01-01T00:00:00Z",
end_date: "2022-01-31T00:00:00Z",
};
const response = await fetch("https://api.vouchery.io/v1/campaigns", {
method: "POST",
headers: {
Authorization: `Bearer ${apiKey}`,
"Content-Type": "application/json",
},
body: JSON.stringify(campaignData),
});
const data = await response.json();
console.log(data);
};
In this example code, we're creating a new campaign in the Vouchery platform. We're passing the API key in the Authorization
header and the campaign data in the request body. The response is then logged to the console.
Get Customer Data
const getCustomers = async () => {
const apiKey = "<your_api_key>";
const response = await fetch("https://api.vouchery.io/v1/customers", {
headers: {
Authorization: `Bearer ${apiKey}`,
},
});
const data = await response.json();
console.log(data);
};
In this example code, we're retrieving customer data from the Vouchery platform. We're passing the API key in the Authorization
header and making a GET request to the https://api.vouchery.io/v1/customers
endpoint. The response is then logged to the console.
Conclusion
In this blog post, we've explored the Vouchery API documentation and looked at some example code in JavaScript. With the Vouchery API, developers can programmatically interact with the Vouchery platform and create and manage voucher campaigns. If you're looking to integrate voucher campaigns into your web or mobile applications, the Vouchery API is a great place to start.